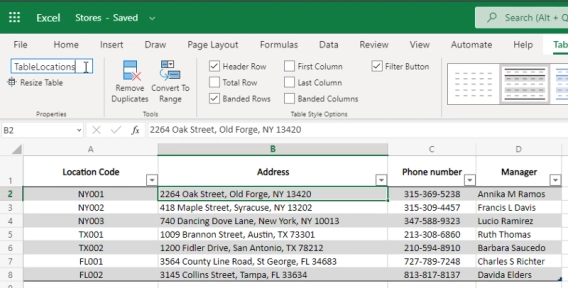
Use OneDrive Excel file as an external source for your Public Web Form fields
Populate fields on your form with data from an Excel file
In this article, I will show you how to get SharePoint list data in Azure Function using Entra ID app and Microsoft Graph API and expose it for anonymous access. I will request the exposed data with JavaScript from a public web form designed with Plumsail Forms for populating options in a dropdown field. Once a user selects an option in this field, I will send another request for getting all column values of the selected item and populating other fields of the same form.
Microsoft Graph API is a unified REST API for programmatic interaction with all Microsoft 365 services—OneDrive, Outlook, Teams, SharePoint, Calendar, Bookings and others. As it provides overwhelming capabilities, you can granulate permissions to the API via Entra ID apps. Say, in this example, I only granted read access to SharePoint data. But if you want to also update items in SharePoint, send emails in Outlook, post messages to Teams, you must provide corresponding permissions.
There are two levels of permissions—Application and Delegated. Application permissions allow the app to get access to requested resources without any limitations and further interactions with users once the permissions are granted by a tenant administrator. The app with delegated access in opposite always acts on behalf of a specific user and is therefore limited to the actions the user is authorized to perform. To get items from SharePoint, I'll use Application permissions.
First of all, we need to register an Entra ID app and grant read access to SharePoint via Microsoft Graph API. To complete this step, you must be a tenant administrator.
Now, we need to provide the app with read access to SharePoint data:
The last step is creating a client secret for our app to use it in Azure Function:
You can deploy a function for getting data from SharePoint Online directly from our GitHub repository. No need to modify source code to specify the app or the data source settings, all of them can be configured via the Function App's environment variables after the deployment:
Once the project is built and deployed, you will see the functions in your Function App. Now, we need to define its environment variables to get access to our SharePoint list.
SharePoint:AzureApp:ClientId
The Application (client) ID of the Entra ID app
SharePoint:AzureApp:ClientSecret
The Client secret of the Entra ID app
SharePoint:AzureApp:Tenant
Your Microsoft 365 tenant, ex.: contoso.onmicrosoft.com
SharePoint:ListData:SiteUrl
The absolute URL of the source site, ex.: https://contoso.sharepoint.com/sites/mysite
SharePoint:ListData:ListName
The name of the source list
Finally, we need to enable Cross-Origin Resource Sharing (CORS) for our app to allow JavaScript requests from our web form:
OK, now we need to copy the SharePoint-ListData function URL to use it in our web form:
Now, we can use the Azure Function for getting SharePoint list data from a public web form created with Plumsail Forms. First, I will populate a dropdown field with SharePoint list items.
I need to copy the field's name to use it in JavaScript for getting access to the field:
Next, take the URL of the SharePoint-ListData function and remove {id} from it. Without this parameter, the function returns all items from the list, should be like this:
https://{your-function-app}.azurewebsites.net/api/sp/items/?code=...
And then, switch to JavaScript editor and paste the code in it:
fd.rendered(() => {
const issueField = fd.field('Issue');
const issueWidget = issueField.widget;
issueWidget.setDataSource({
transport: {
read: '-- URL of SharePoint-ListData (without {id}) --'
},
schema: {
// transforming results from Microsoft Graph API to the list of flat objects: {id, title}
data: items => {
return items.map(i => ({id: i.id, title: i.fields.additionalData.Title}));
}
}
});
// the Issue dropdown will show the Title, but its value will be the ID.
issueWidget.setOptions({dataTextField: 'title', dataValueField: 'id'});
});
Now, after rendering the form, expand the dropdown and you will find all SharePoint items here:
Next step is populating other fields based on the selection in the dropdown field. Get back to the JavaScript editor and add the function for requesting a selected SharePoint item by its id and populating fields from its properties:
async function populateIssueDetails(itemId) {
// Replace {id} in SharePoint-ListData URL with itemId
const response = await fetch(`https://{your-function-app}.azurewebsites.net/api/sp/items/${itemId}?code=...`);
if (response.ok) {
const item = await response.json();
fd.field('Description').value = item.Description;
fd.field('Priority').value = item.Priority;
fd.field('Status').value = item.Status;
fd.field('DateReported').value = item.DateReported;
} else {
fd.field('Description').value =
fd.field('Priority').value =
fd.field('Status').value =
fd.field('DateReported').value = null;
}
}
Finally, we need to call this function when a user changes the selection:
fd.rendered(() => {
const issueField = fd.field('Issue');
issueField.$on('change', v => populateIssueDetails(v));
});
Simple, isn't it?
If you also need to modify data in SharePoint after submitting a form, you can do it either via Power Automate or the same Azure Function with following adjustments:
Here, I demonstrated how to use data from SharePoint in a public web form. The same way you can retrieve data from other SharePoint lists, Microsoft 365 apps, or any services which expose public API.
Of course, this is a simple example and might not fully meet your needs. It's just to demonstrate how to integrate public forms with SharePoint Online via Azure Functions and Microsoft Graph API. Feel free to share your specific use cases in our community, and we'll be happy to address them in future posts.