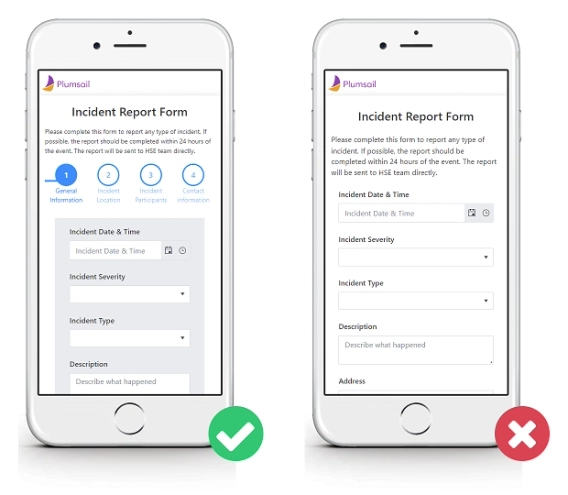
How to design Mobile-Friendly Online Forms
Elevate your web forms for mobile era! Follow these 7 simple tips to create mobile-friendly online forms that are responsive and easy to complete.
If you have a large form and don't want to scare users away with too many fields, you should consider hiding some fields by default and showing them only when necessary.
In Plumsail Public Web Forms, you can create interactive forms that response dynamically to input data using JavaScript code:
Here we will review some examples that you can reuse in your form.
The simplest case is to show or hide a field based on a single field value.
Suppose that users can choose whether they want to receive SMS notifications. And if they do, they must enter a phone number. This way, you keep the form clean and make it more interactive.
You add the code to show or hide the Phone field dynamically based on a user's answer:
function showHidePhone() {
if (fd.field('Notifications').value === 'Yes') {
fd.field('Phone').hidden = false;
} else {
fd.field('Phone').hidden = true;
}
}
fd.rendered(function() {
//call function on field change
fd.field('Notifications').$on('change', showHidePhone);
//call function on form load
showHidePhone();
});
The next level is to show or hide the field based on multiple field values. You can create complex conditions, choosing whether to show a field if all conditions are met or if one of the conditions is true.
Let me give you an example. There is a health screening form. When users have no vaccination against covid-19 and come from abroad, they must schedule a test date:
We add conditional logic to show the Test Date field only when a user answers yes to both questions:
function showHideDate() {
if (fd.field('Vaccinated').value === 'No' && fd.field('Traveled').value === 'Yes') {
fd.field('Date').hidden = false;
} else {
fd.field('Date').hidden = true;
}
}
fd.rendered(function() {
//call function on field change
fd.field('Vaccinated').$on('change', showHideDate);
fd.field('Traveled').$on('change', showHideDate);
//call function on form load
showHideDate();
});
Another example is when you want to verify that the field value is valid before allowing a user to move forward.
Assume you want users to enter a valid email before they can proceed to fill out the form.
You can do that by adding a few lines of the code:
function showHideSubscriptionPlan() {
if (fd.field('Email').validate() && fd.field('Email').value) {
fd.field('SubscriptionPlan').hidden = false;
} else {
fd.field('SubscriptionPlan').hidden = true;
}
}
fd.rendered(function() {
//call function on field change
fd.field('Email').$on('change', showHideSubscriptionPlan);
//call function on form load
showHideSubscriptionPlan();
});
What if you want to show more than one field at a time? You can do that too.
To give you an idea, users can choose from several delivery options: to the pickup-point, the company office, or courier delivery. When choosing courier delivery user must see the address fields, such as street, city, state, and zip code:
You simply add a common class to address fields. Then, you can show/hide them all at once using jQuery method:
function showHideAddress() {
if (fd.field('DeliveryType').value === 'courier delivery') {
$('.address').show();
} else {
$('.address').hide();
}
}
fd.rendered(function() {
//call function on field change
fd.field('DeliveryType').$on('change', showHideAddress);
//call function on form load
showHideAddress();
});
In some cases, it is necessary to show the certain number of fields, depending on user preferences.
Let's look at a cake order form. Users can select the number of layers they want and choose different flavors for each layer. When a user selects the number of layers, the corresponding number of fields are shown:
To do so, we add the code to the form:
function showHideLayers() {
//hide layer fields
fd.field('Layer1Flavor').hidden = true;
fd.field('Layer2Flavor').hidden = true;
fd.field('Layer3Flavor').hidden = true;
//show layer fields depending on the selected value
if (fd.field('NumberOfLayers').value == '1') {
fd.field('Layer1Flavor').hidden = false;
} else if (fd.field('NumberOfLayers').value == '2') {
fd.field('Layer1Flavor').hidden = false;
fd.field('Layer2Flavor').hidden = false;
} else if (fd.field('NumberOfLayers').value == '3') {
fd.field('Layer1Flavor').hidden = false;
fd.field('Layer2Flavor').hidden = false;
fd.field('Layer3Flavor').hidden = false;
}
}
fd.rendered(function() {
//call function on field change
fd.field('NumberOfLayers').$on('change', showHideLayers);
//call function on form load
showHideLayers();
});
Put these tips into practice today. Create a Plumsail account and design a mobile-friendly form for your business needs.